Hello everybody! In this post I’m going to show you how to install third party MSI files using InstallAware. We’ll achieve this functionality using the (Un)Install MSI Setup and File Bag MSIcode commands.
First, what does the File Bag command do? Think of it as an extended support files mechanism. While support files (also called Creatives in the InstallAware IDE’s visual designers) are a great way to add temporary files to your setup, they lack the ability to recurse into multiple folders. Support files also cannot be placed inside a web media block – they always go inside the main setup.exe file. So File Bag is essentially a fix for these two problems – this command lets you add temporary files to your setup which are only available while setup is running (and not permanently installed on the end-user system), is web media block friendly, and also supports recursion.
Next, what does (Un)Install MSI Setup do? As the name implies, it can install or uninstall an MSI based setup. The beauty of this command, as opposed to using another built-in command like Run Program to install third party MSI files, is that it captures native progress as well as the final error/success state of the installation, along with any textual error description text if applicable. This makes your setup look more professional: even the progress of external setups will be displayed in your own custom dialogs at install time, and you can provide very effective error handling and troubleshooting.
Now that we’ve gotten the preliminaries out of the way, lets get to work. A good example of to use the File Bag command is in our own pre-built setup script for the Microsoft .NET Framework 3.0. You can add this runtime to your setup and then switch to the MSIcode view to investigate how we’ve used the command.
The File Bag MSIcode statement:

And the File Bag command window:
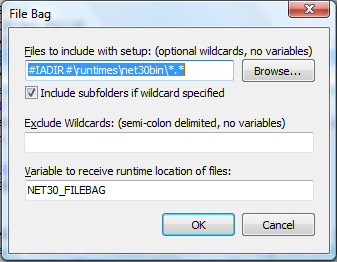
The pre-defined compiler variable #IADIR# simply returns InstallAware‘s main installation folder, which is the parent folder of the setup files for the .NET 3 Framework. In this field, you can enter any path you like, or just a single file name – as long as it points to the file(s) you want to add to your setup as a temporary resource.
The other interesting field in this command window is the NET30_FILEBAG variable. You may enter any custom variable in this field (as long as you pre-initialize it using the Set Variable command to an empty value). You should always provide a variable in this field, because the temporary location where File Bag makes your “bagged files” available at runtime will always change from machine to machine and setup to setup. So unless you provide a variable in this field, you won’t be able to access your bagged files at runtime, which beats the whole purpose of using File Bag in the first place!
Next lets take a look at the command window for the (Un)Install MSI Setup plug-in.
The Action tab indicates the installation/uninstallation mode and passes additional command line parameters to setup:
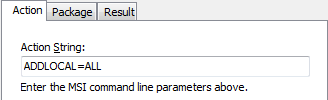
When installing an MSI file, you typically enter ADDLOCAL=ALL here, to install all of the features that are provided with the MSI. When uninstalling, you typically enter REMOVE=ALL. You are free to set any other MSI properties using this field, to install precisely those features that you require, or perform a customized MSI installation.
The Package tab specifies the MSI package to use, by filename (used when installing) or by package GUID (used when uninstalling):
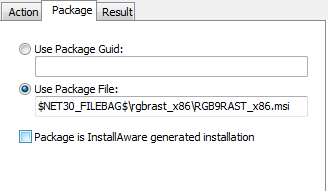
When installing, you’ll want to specify the full path to the third party MSI file. The path will always be composed of the variable populated by File Bag, followed by the subfolder (if any) and the MSI file name. When uninstalling, you may again reference the MSI file by its full file path; alternately you may simply reference it by its unique package GUID (the GUID may be found by opening up your MSI package in Orca, navigating to the Property table, and copying the full value, including the squiggly parenthesis, corresponding to the ProductCode property). Using the GUID can be more advantageous when uninstalling, since you won’t need to provide access to the MSI file (often inconvenient to do at uninstall time – you hardly want to prompt your end-users for a setup CD when they’re just trying to remove your product).
Here’s an MSIcode snippet that shows the proper placement of the (Un)Install MSI Setup command for use at uninstall time:

And this is how the Action tab would look for the command when uninstalling (again, feel free to add additional properties):
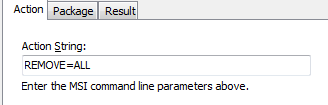
So now, you know all you need to add temporary files to your setup, and to run third party MSIs, even natively capturing their native setup progress, to install or remove them 🙂
One last thing worthy of mention is the Setup Decompressor. This is a very handy tool that InstallAware originally built for Microsoft in 2005, to optimize the compressibility of their then-current .NET 1.1 Framework. This tool works with MSI (and a few other file types) and decompresses their data. However, it doesn’t just “extract” data like unzipping a ZIP file. What it does is, it first extracts the data, then re-inserts it back into the original file, in unmodified form – only uncompressed this time (think of it like re-creating a ZIP file with “stored” compression, so the files inside the ZIP archive are not compressed but just stored as-is).
Why would anybody want to do this? Data Compression 101: No matter how good a compression algorithm is, it cannot recompress data that has been compressed before. So for example, lets say you have a 10 MB file, and when you ZIP it, it goes down to 5 MB, and when you CAB it, it goes down to 3 MB…you might think that when you CAB the 5 MB ZIP, it would again come down to 3 MB…but that’s not the case. You still get a 5 MB CAB – because the 5 MB ZIP has already eliminated redundancies in the original uncompressed data stream (even though it did a worse job at it than the CAB).
InstallAware‘s compression algorithm is far better than the compression used in MSI files, but if you feed it pre-compressed data (such as “off-the-shelf” MSI files, which are always compressed using CAB), it won’t be able to reduce file sizes any further. For this reason, you want to run the Setup Decompressor on your MSI files before packaging your setup with InstallAware. Since Setup Decompressor is non-destructive, it will never harm your MSI files or change the files they contain in any way – other than reversing their compression. And this way, InstallAware is free to work miracles in compressing your MSI files!
Just take a look at .NET Framework sizes, when packaged natively (as by Microsoft) and with InstallAware (after pre-processing by the Setup Decompressor). Typical size savings are 40% over the already compressed size of the native Microsoft files, and sometimes as high as 67% (as in the case of the .NET Framework 1.1 with SP1 inlined). You’ll be pleased to know that the Setup Decompressor also works with other file types – such as CAB, MSM, MSP, and even some kinds of EXE files. Just try it out!
To open the Setup Decompressor tool, click the Decompress button in the Tools group on the Design tab of the InstallAware IDE:
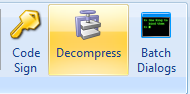
All you have to after this is click the Browse button and select the MSI file you want to decompress. Click Decompress and the fully automated Setup Decompressor gets to work for you – and provides live status update as it investigates the various archives and streams inside your MSI file. I also recommend checking the Backup original file option – just in case you run into a bug that we haven’t encountered yet 😉
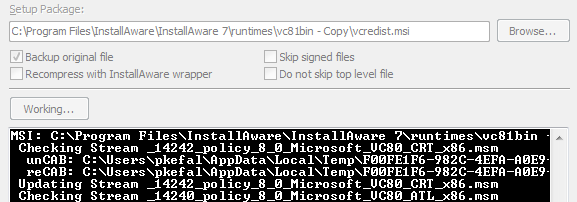
So, that’s it for now. In my next post, we’ll cover a related topic, “How to run a third party EXE using InstallAware“. Until then!
Thank you,
Panagiotis Kefalidis
Software Design Team Lead
InstallAware Software Corporation